- Cmakelists Add Executable
- Add_executable Example In Linux
- Cmake Add Executable
- Cmake Add_executable Imported Example
This tutorial aims to get you up and running with GoogleTest using CMake. Ifyou're using GoogleTest for the first time or need a refresher, we recommendthis tutorial as a starting point. If your project uses Bazel, see theQuickstart for Bazel instead.
Prerequisites
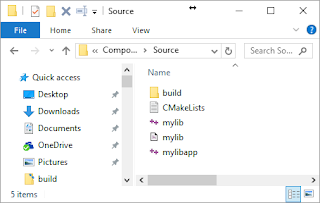
This example action server generates a Fibonacci sequence, the goal is the order of the sequence, the feedback is the sequence as it is computed, and the result is the final sequence. Tutorial Level: BEGINNER Next Tutorial: Write a simple action client. Example: linkdirectories(/mylibs) Please see this cmake thread to see a detailed example of using targetlinklibraries over linkdirectories. Executable Targets. To specify an executable target that must be built, we must use the addexecutable CMake function. Addexecutable(myProgram src/main.cpp src/somefile.cpp src/anotherfile.cpp). Addexecutable (exampleenclave example.c examplet.c) targetlinklibraries (exampleenclave openenclave::oeenclave openenclave::oelibc) Technically, the openenclave::oelibc dependency is optional. That is, if you don't use anything from the C library (such as #include etc.), you can exclude it, but this is very unlikely.
To complete this tutorial, you'll need:
- A compatible operating system (e.g. Linux, macOS, Windows).
- A compatible C++ compiler that supports at least C++11.
- CMake and a compatible build tool for building theproject.
- Compatible build tools includeMake,Ninja, and others - seeCMake Generatorsfor more information.
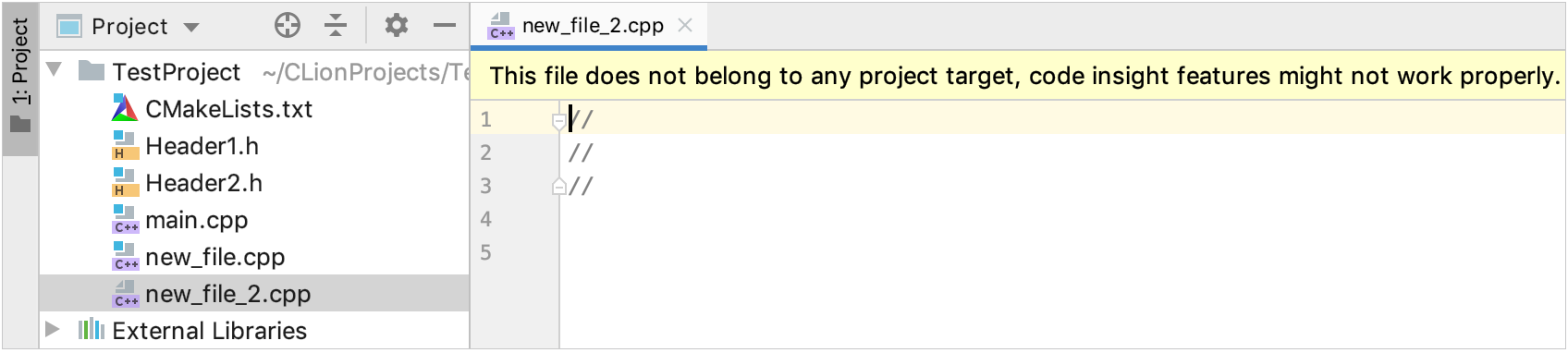
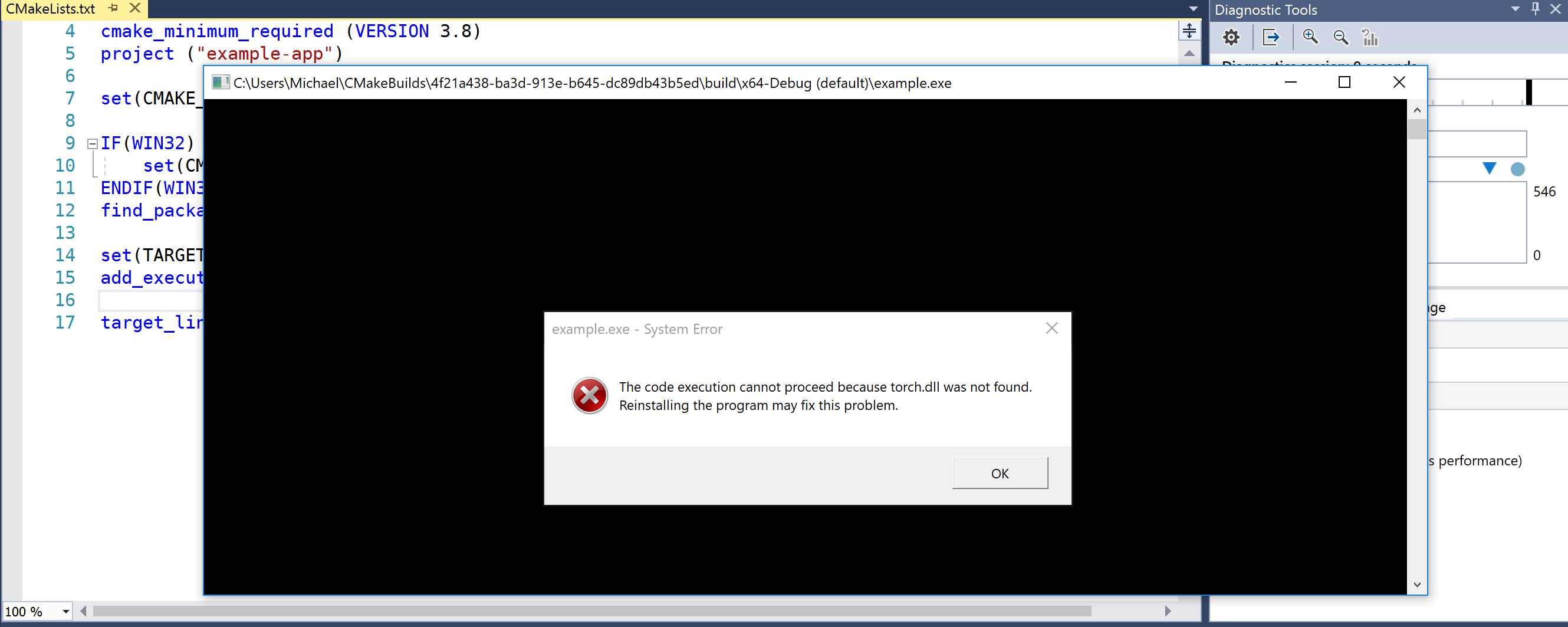
See Supported Platforms for more information about platformscompatible with GoogleTest.
If you don't already have CMake installed, see theCMake installation guide.
Note: The terminal commands in this tutorial show a Unix shell prompt, but thecommands work on the Windows command line as well.
Set up a project
With addexecutable (app main.cpp) a build target app is created, which will invoke the configured compiler with some default flags for the current setting to compile an executable app from the given source file main.cpp. Command Line (In-Source-Build, not recommended) cmake. cmake -build. Creating a Bash File. Rhetorical precis template doc. The first step is to create a new text file with.sh extension using the following.
CMake uses a file named CMakeLists.txt
to configure the build system for aproject. You'll use this file to set up your project and declare a dependency onGoogleTest.
First, create a directory for your project:
Next, you'll create the CMakeLists.txt
file and declare a dependency onGoogleTest. There are many ways to express dependencies in the CMake ecosystem;in this quickstart, you'll use theFetchContent
CMake module.To do this, in your project directory (my_project
), create a file namedCMakeLists.txt
with the following contents:
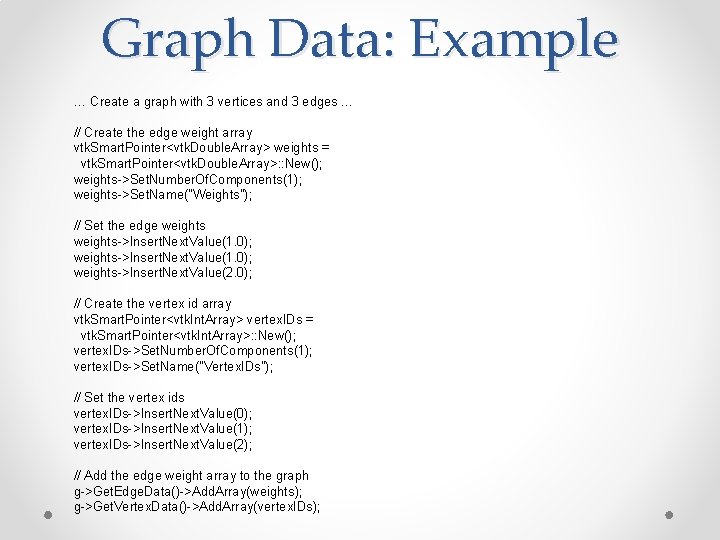
This example action server generates a Fibonacci sequence, the goal is the order of the sequence, the feedback is the sequence as it is computed, and the result is the final sequence. Tutorial Level: BEGINNER Next Tutorial: Write a simple action client. Example: linkdirectories(/mylibs) Please see this cmake thread to see a detailed example of using targetlinklibraries over linkdirectories. Executable Targets. To specify an executable target that must be built, we must use the addexecutable CMake function. Addexecutable(myProgram src/main.cpp src/somefile.cpp src/anotherfile.cpp). Addexecutable (exampleenclave example.c examplet.c) targetlinklibraries (exampleenclave openenclave::oeenclave openenclave::oelibc) Technically, the openenclave::oelibc dependency is optional. That is, if you don't use anything from the C library (such as #include etc.), you can exclude it, but this is very unlikely.
To complete this tutorial, you'll need:
- A compatible operating system (e.g. Linux, macOS, Windows).
- A compatible C++ compiler that supports at least C++11.
- CMake and a compatible build tool for building theproject.
- Compatible build tools includeMake,Ninja, and others - seeCMake Generatorsfor more information.
See Supported Platforms for more information about platformscompatible with GoogleTest.
If you don't already have CMake installed, see theCMake installation guide.
Note: The terminal commands in this tutorial show a Unix shell prompt, but thecommands work on the Windows command line as well.
Set up a project
With addexecutable (app main.cpp) a build target app is created, which will invoke the configured compiler with some default flags for the current setting to compile an executable app from the given source file main.cpp. Command Line (In-Source-Build, not recommended) cmake. cmake -build. Creating a Bash File. Rhetorical precis template doc. The first step is to create a new text file with.sh extension using the following.
CMake uses a file named CMakeLists.txt
to configure the build system for aproject. You'll use this file to set up your project and declare a dependency onGoogleTest.
First, create a directory for your project:
Next, you'll create the CMakeLists.txt
file and declare a dependency onGoogleTest. There are many ways to express dependencies in the CMake ecosystem;in this quickstart, you'll use theFetchContent
CMake module.To do this, in your project directory (my_project
), create a file namedCMakeLists.txt
with the following contents:
The above configuration declares a dependency on GoogleTest which is downloadedfrom GitHub. In the above example, 609281088cfefc76f9d0ce82e1ff6c30cc3591e5
isthe Git commit hash of the GoogleTest version to use; we recommend updating thehash often to point to the latest version.
Cmakelists Add Executable
For more information about how to create CMakeLists.txt
files, see theCMake Tutorial.
Create and run a binary
With GoogleTest declared as a dependency, you can use GoogleTest code withinyour own project.
As an example, create a file named hello_test.cc
in your my_project
directory with the following contents:
Add_executable Example In Linux
GoogleTest provides assertions that you use to test thebehavior of your code. The above sample includes the main GoogleTest header fileand demonstrates some basic assertions.
To build the code, add the following to the end of your CMakeLists.txt
file:
The above configuration enables testing in CMake, declares the C++ test binaryyou want to build (hello_test
), and links it to GoogleTest (gtest_main
). Thelast two lines enable CMake's test runner to discover the tests included in thebinary, using theGoogleTest
CMake module.
Now you can build and run your test:
Congratulations! You've successfully built and run a test binary usingGoogleTest.
Cmake Add Executable
Next steps
Cmake Add_executable Imported Example
- Check out the Primer to start learning how to write simpletests.
- See the code samples for more examples showing how to use avariety of GoogleTest features.